cozmo.annotate¶
Camera image annotation.
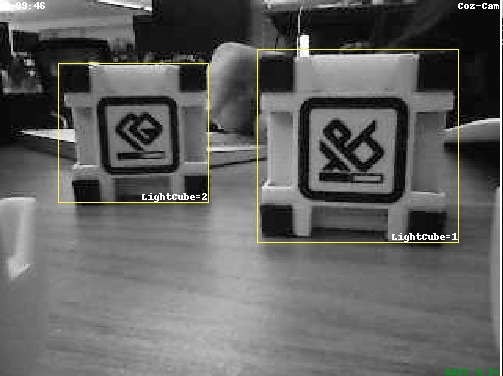
This module defines an ImageAnnotator
class used by
cozmo.world.World
to add annotations to camera images received by Cozmo.
This can include the location of cubes, faces and pets that Cozmo currently sees, along with user-defined custom annotations.
The ImageAnnotator instance can be accessed as
cozmo.world.World.image_annotator
.
Functions
add_img_box_to_image (image, box, color[, text]) |
Draw a box on an image and optionally add text. |
add_polygon_to_image (image, poly_points, …) |
Draw a polygon on an image |
annotator (f) |
A decorator for converting a regular function/method into an Annotator. |
Classes
Annotator (img_annotator[, priority]) |
Annotation base class |
FaceAnnotator (img_annotator[, box_color]) |
Adds annotations of currently detected faces to a camera image. |
ImageAnnotator (world, **kw) |
ImageAnnotator applies annotations to the camera image received from the robot. |
ImageText (text[, position, align, color, …]) |
ImageText represents some text that can be applied to an image. |
ObjectAnnotator (img_annotator[, object_colors]) |
Adds object annotations to an Image. |
PetAnnotator (img_annotator[, box_color]) |
Adds annotations of currently detected pets to a camera image. |
TextAnnotator (img_annotator, text) |
Adds simple text annotations to a camera image. |
-
cozmo.annotate.
TOP_LEFT
= 5¶ Top left position
-
cozmo.annotate.
TOP_RIGHT
= 6¶ Top right position
-
cozmo.annotate.
BOTTOM_LEFT
= 9¶ Bottom left position
-
cozmo.annotate.
BOTTOM_RIGHT
= 10¶ Bottom right position
-
cozmo.annotate.
RESAMPLE_MODE_NEAREST
= 0¶ Fastest resampling mode, use nearest pixel
-
cozmo.annotate.
RESAMPLE_MODE_BILINEAR
= 2¶ Slower, but smoother, resampling mode - linear interpolation from 2x2 grid of pixels
-
class
cozmo.annotate.
ImageText
(text, position=10, align='left', color='white', font=None, line_spacing=3, outline_color=None, full_outline=True)¶ ImageText represents some text that can be applied to an image.
The class allows the text to be placed at various positions inside a bounding box within the image itself.
Parameters: - text (string) – The text to display; may contain newlines
- position (int) – Where on the screen to render the text - A constant such at TOP_LEFT or BOTTOM_RIGHT
- align (string) – Text alignment for multi-line strings
- color (string) – Color to use for the text - see
PIL.ImageColor
- font (
PIL.ImageFont
) – Font to use (None for a default font) - line_spacing (int) – The vertical spacing for multi-line strings
- outline_color (string) – Color to use for the outline - see
PIL.ImageColor
- use None for no outline. - full_outline (bool) – True if the outline should surround the text, otherwise a cheaper drop-shadow is displayed. Only relevant if outline_color is specified.
-
render
(draw, bounds)¶ Renders the text onto an image within the specified bounding box.
Parameters: - draw (
PIL.ImageDraw.ImageDraw
) – The drawable surface to write on - bounds (tuple of int) – (top_left_x, top_left_y, bottom_right_x, bottom_right_y): bounding box
Returns: The same
PIL.ImageDraw.ImageDraw
object as was passed-in with text applied.- draw (
-
class
cozmo.annotate.
Annotator
(img_annotator, priority=None)¶ Annotation base class
Subclasses of Annotator handle applying a single annotation to an image.
-
apply
(image, scale)¶ Applies the annotation to the image.
-
img_annotator
= None¶ The object managing camera annotations
Type: ImageAnnotator
-
-
class
cozmo.annotate.
ObjectAnnotator
(img_annotator, object_colors=None)¶ Adds object annotations to an Image.
This handles
cozmo.objects.LightCube
objects as well as custom objects.-
apply
(image, scale)¶ Applies the annotation to the image.
-
label_for_obj
(obj)¶ Fetch a label to display for the object.
Override or replace to customize.
-
-
class
cozmo.annotate.
FaceAnnotator
(img_annotator, box_color=None)¶ Adds annotations of currently detected faces to a camera image.
This handles the display of
cozmo.faces.Face
objects.-
apply
(image, scale)¶ Applies the annotation to the image.
-
label_for_face
(obj)¶ Fetch a label to display for the face.
Override or replace to customize.
-
-
class
cozmo.annotate.
PetAnnotator
(img_annotator, box_color=None)¶ Adds annotations of currently detected pets to a camera image.
This handles the display of
cozmo.pets.Pet
objects.-
apply
(image, scale)¶ Applies the annotation to the image.
-
label_for_pet
(obj)¶ Fetch a label to display for the pet.
Override or replace to customize.
-
-
class
cozmo.annotate.
TextAnnotator
(img_annotator, text)¶ Adds simple text annotations to a camera image.
-
apply
(image, scale)¶ Applies the annotation to the image.
-
-
class
cozmo.annotate.
ImageAnnotator
(world, **kw)¶ ImageAnnotator applies annotations to the camera image received from the robot.
This is instantiated by
cozmo.world.World
and is accessible ascozmo.world.World.image_annotator
.By default it defines three active annotators named
objects
,faces
andpets
.The
objects
annotator adds a box around each object (such as light cubes) that Cozmo can see. Thefaces
annotator adds a box around each person’s face that Cozmo can recognize. Thepets
annotator adds a box around each pet face that Cozmo can recognize.Custom annotations can be defined by calling
add_annotator()
with a name of your choosing and an instance of aAnnotator
subclass, or use a regular function wrapped with theannotator()
decorator.Individual annotations can be disabled and re-enabled using the
disable_annotator()
andenable_annotator()
methods.All annotations can be disabled by setting the
annotation_enabled
property to False.E.g. to disable face annotations, call
coz.world.image_annotator.disable_annotator('faces')
Annotators each have a priority number associated with them. Annotators with a larger priority number are rendered first and may be overdrawn by those with a lower/smaller priority number.
-
add_annotator
(name, annotator)¶ Adds a new annotator for display.
Annotators are enabled by default.
Parameters: - name (string) – An arbitrary name for the annotator; must not already be defined
- annotator (
Annotator
or callable) – The annotator to add may either by an instance of Annotator, or a factory callable that will return an instance of Annotator. The callable will be called with an ImageAnnotator instance as its first argument.
Raises: ValueError
if the annotator is already defined.
-
add_static_text
(name, text, color='white', position=5)¶ Add some static text to annotated images.
This is a convenience method to create a
TextAnnnotator
and add it to the image.Parameters: - name (string) – An arbitrary name for the annotator; must not already be defined
- text (str or
ImageText
instance) – The text to display may be a plain string, or an ImageText instance - color (string) – Used if text is a string; defaults to white
- position (int) – Used if text is a string; defaults to TOP_LEFT
-
annotate_image
(image, scale=None, fit_size=None, resample_mode=0)¶ Called by
World
to annotate camera images.Parameters: - image (
PIL.Image.Image
) – The image to annotate - scale (float) – If set then the base image will be scaled by the supplied multiplier. Cannot be combined with fit_size
- fit_size (tuple of int) – If set, then scale the image to fit inside the supplied (width, height) dimensions. The original aspect ratio will be preserved. Cannot be combined with scale.
- resample_mode (int) – The resampling mode to use when scaling the
image. Should be either
RESAMPLE_MODE_NEAREST
(fast) orRESAMPLE_MODE_BILINEAR
(slower, but smoother).
Returns: - image (
-
annotation_enabled
= None¶ If this attribute is set to false, the
annotate_image()
method will continue to provide a scaled image, but will not apply any annotations.
-
disable_annotator
(name)¶ Disable a named annotator.
Leaves the annotator as registered, but does not include its output in the annotated image.
Parameters: name (string) – The name of the annotator to disable
-
enable_annotator
(name)¶ Enabled a named annotator.
(re)enable an annotator if it was previously disabled.
Parameters: name (string) – The name of the annotator to enable
-
get_annotator
(name)¶ Return a named annotator.
Parameters: name (string) – The name of the annotator to return Raises: KeyError if the annotator isn’t registered
-
remove_annotator
(name)¶ Remove an annotator.
Parameters: name (string) – The name of the annotator to remove as passed to add_annotator()
.Raises: KeyError if the annotator isn’t registered
-
world
= None¶ World object that created the annotator.
Type: cozmo.world.World
-
-
cozmo.annotate.
add_img_box_to_image
(image, box, color, text=None)¶ Draw a box on an image and optionally add text.
This will draw the outline of a rectangle to the passed in image in the specified color and optionally add one or more pieces of text along the inside edge of the rectangle.
Parameters: - image (
PIL.Image.Image
) – The image to draw on - box (
cozmo.util.ImageBox
) – The ImageBox defining the rectangle to draw - color (string) – A color string suitable for use with PIL - see
PIL.ImageColor
- text (instance or iterable of
ImageText
) – The text to display - may be a single ImageText instance, or any iterable (eg a list of ImageText instances) to display multiple pieces of text.
- image (
-
cozmo.annotate.
add_polygon_to_image
(image, poly_points, scale, line_color, fill_color=None)¶ Draw a polygon on an image
This will draw a polygon on the passed-in image in the specified colors and scale.
Parameters: - image (
PIL.Image.Image
) – The image to draw on - poly_points – A sequence of points representing the polygon, where each point has float members (x, y)
- scale (float) – Scale to multiply each point to match the image scaling
- line_color (string) – The color for the outline of the polygon. The string value
must be a color string suitable for use with PIL - see
PIL.ImageColor
- fill_color (string) – The color for the inside of the polygon. The string value
must be a color string suitable for use with PIL - see
PIL.ImageColor
- image (
-
cozmo.annotate.
annotator
(f)¶ A decorator for converting a regular function/method into an Annotator.
The wrapped function should have a signature of
(image, scale, img_annotator=None, world=None, **kw)